shtfxdh が 2024年10月29日17時23分20秒 に編集
コメント無し
本文の変更
# 概要 ・天気の情報、ニュースなどを送ります。 ・毎日指定した時間にLINEに送られるようにしています。 ・天気に画像を送ります。 # 詳細 RaspberryPiを使用して天気情報などをlinenotifyを使用して毎日指定した時間に送ります。 天気情報の:Yahoo天気から高知県中部の天気情報を取得し、メッセージ形式に整えます。 ニュースの取得:Yahooニュースからトップ5の見出しを取得し、メッセージ形式に整えます。 LINE通知の送信:天気情報とニュースをLINEに送信します。天気に応じた画像も添付します。 # イメージ図 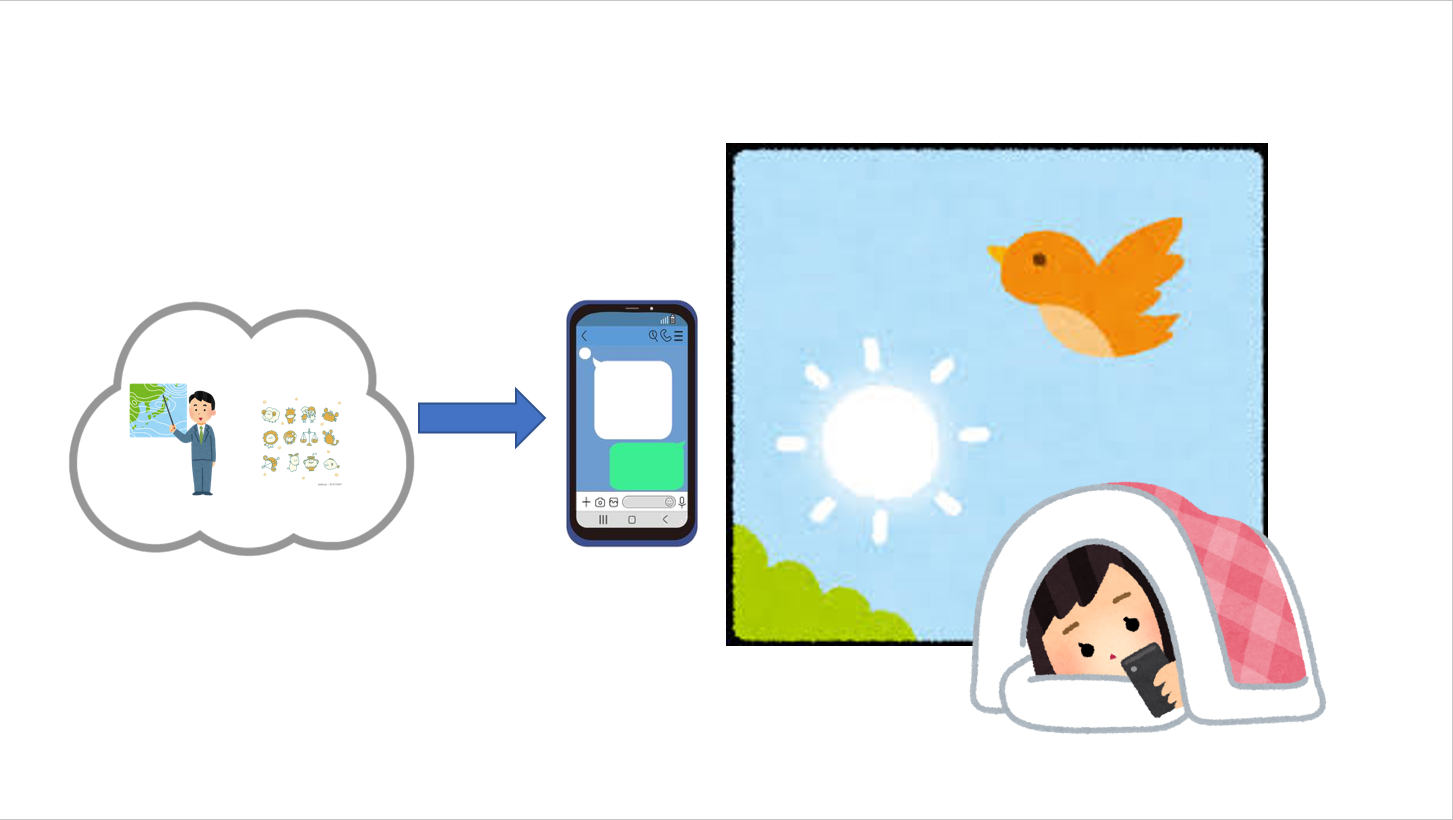
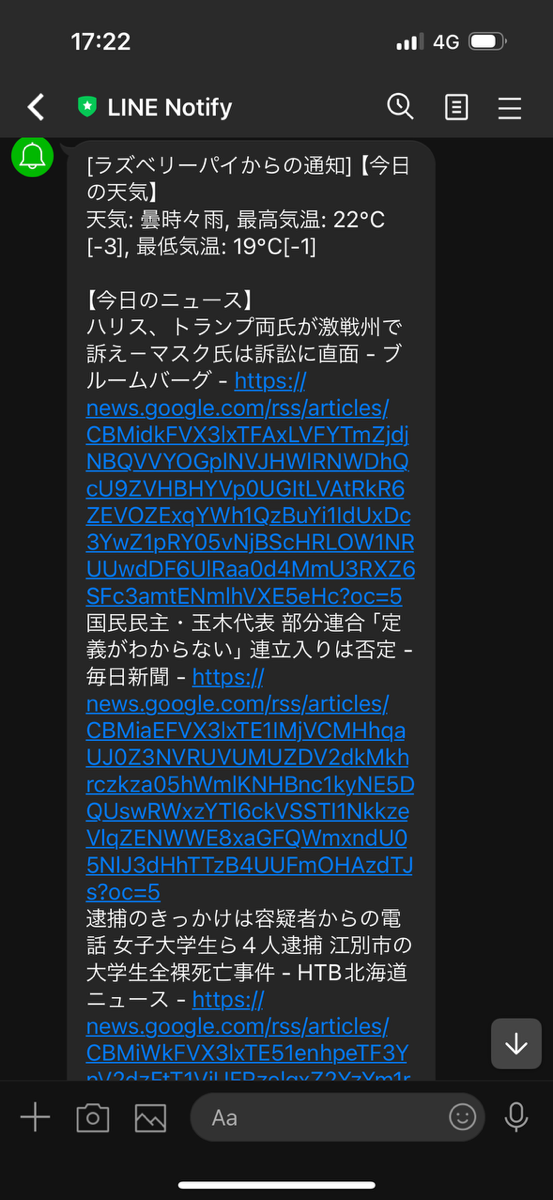
# 使用した部品 今回の製作で使用した部品の紹介 ・RaspberryPI 4 ・スマートフォン # 参考文献 ・LINEでOpenWeatherMapの天気情報を通知する仕組みの解説 https://kaizen-factory.jp/line_notify_openwheathermap/ ・【仕事の自動化】PythonでLINEを操作。メッセージや画像を自分へ送信しよう! https://kino-code.com/pythonline/
```arduino:プログラム import requests from bs4 import BeautifulSoup import schedule import time import feedparser # RSSフィードを解析するためのライブラリ # LINE Notifyの設定 line_notify_token = 'sI2F3FCBxGOtyOui8CkkL7QdOUiMKZ9WF1f41mUyyaS' # LINE Notifyの正しいアクセストークンを設定 line_notify_api = 'https://notify-api.line.me/api/notify' # Yahoo!天気のWebページから天気情報を取得する関数 def get_weather(): try: url = 'https://weather.yahoo.co.jp/weather/jp/39/7410.html' # 高知県中部の天気情報 response = requests.get(url) response.raise_for_status() # HTTPリクエストで問題が発生した場合例外を送出 soup = BeautifulSoup(response.content, 'html.parser') # 天気概要を取得する forecast = soup.find(class_='forecastCity') # 天気のメイン情報が入っている要素 if not forecast: return "天気情報の取得に失敗しました" # 天気概要のテキストを取得 weather_info = forecast.find('p', class_='pict').text.strip() if forecast.find('p', class_='pict') else '天気情報なし' # 最高気温と最低気温を取得 temp_max = forecast.find('li', class_='high').text.strip() if forecast.find('li', class_='high') else '最高気温情報なし' temp_min = forecast.find('li', class_='low').text.strip() if forecast.find('li', class_='low') else '最低気温情報なし' return f"天気: {weather_info}, 最高気温: {temp_max}, 最低気温: {temp_min}" except Exception as e: return f"天気情報の取得に失敗しました: {e}" # Google News RSSからニュース情報を取得する関数 def get_news(): try: rss_url = "https://news.google.com/rss?hl=ja&gl=JP&ceid=JP:ja" # 日本のGoogle NewsのRSS news_feed = feedparser.parse(rss_url) top_articles = news_feed['entries'][:5] # 最新の5件のニュースを取得 news_list = [f"{entry['title']} - {entry['link']}" for entry in top_articles] return '\n'.join(news_list) except Exception as e: return f"ニュースの取得に失敗しました: {e}" def send_notify(message, weather): if '晴れ' in weather: image = '/home/pi/sunny.png' elif '曇り' in weather: image = '/home/pi/cloudy.png' elif '雨' in weather: image = '/home/pi/rainy.jpg' else: image = '/home/pi/default.jpg' # LINE Notifyで通知を送信する関数 def send_line_notify(message): headers = {'Authorization': 'Bearer ' + line_notify_token} data = {'message': message} try: response = requests.post(line_notify_api, headers=headers, data=data) response.raise_for_status() # リクエストが失敗した場合例外を発生 print("通知を送信しました") except requests.exceptions.RequestException as e: print(f"通知の送信に失敗しました: {e}") # ジョブを実行する関数 def job(): weather_message, weather_status = get_weather() news_message = get_news() message = f"【今日の天気】\n{weather_message}\n\n【今日のニュース】\n{news_message}" send_line_notify(message) send_notify(message, weather_status) # 毎日指定した時間にジョブを実行(例: 午前7時に実行) schedule.every().day.at("16:42").do(job) # 時間を希望に合わせて設定 # スケジュールをループして監視 while True: schedule.run_pending() time.sleep(1) ```