komatsu が 2024年10月24日14時06分40秒 に編集
コメント無し
本文の変更
概要 - 部屋の温度が決めた値を超えたとき、Lineで現在の部屋の温度、湿度を送信し、エアコンを操作することで熱中症を対策する 毎朝、その日の最高気温、最低気温、天気をLineで送信する イメージ - 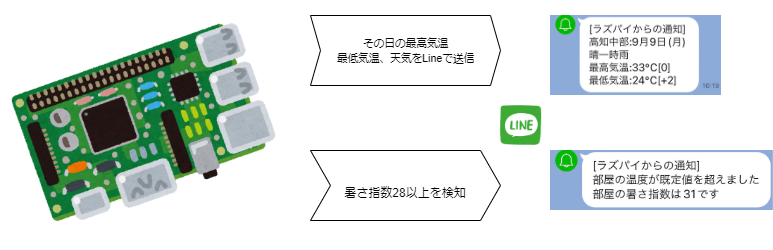 アイデアの詳細 - 部屋の温度はBME280で温度・湿度を用いて暑さ指数(WBGT)を測定し、規定値(28以上)になるとLineを送信する その日の最高気温、最低気温、天気をyahooから取得し、Lineに送信する # ソースコード ``` import tkinter import numpy as np from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg, NavigationToolbar2Tk from matplotlib.figure import Figure import matplotlib.animation as animation import RPi.GPIO as GPIO import smbus2 import bme280 import lcd_st7032 from datetime import datetime import time import sys import io import requests from bs4 import BeautifulSoup import numpy.random as random import matplotlib.pyplot as plt port = 1 address = 0x76 bus = smbus2.SMBus(port) date_t = []; date_h = []; date_p = []; X_LIMIT = 100 RESOLUSION = 0.2 line_notify_token = 'Lineトークン' line_notify_api = 'https://notify-api.line.me/api/notify' # BME280が接続されているかを確認 try: bus.read_byte(address) except: print('BME280が接続されていません') sys.exit() # BME280を初期化 calibration_params = bme280.load_calibration_params(bus, address) # START print("Start check2.py") print("(Ctrl-C to EXIT)") print() class GUI: cntt = 0 def __init__(self): data = bme280.sample(bus, address, calibration_params) t = data.temperature h = data.humidity p = data.pressure self.data_t = np.zeros(int(X_LIMIT / RESOLUSION), dtype=int) self.data_h = np.zeros(int(X_LIMIT / RESOLUSION), dtype=int) self.data_p = np.zeros(int(X_LIMIT / RESOLUSION), dtype=int) self.root = tkinter.Tk() self.root.wm_title("Embedding in Tk anim") self.fig = Figure() canvas = FigureCanvasTkAgg(self.fig, master=self.root) x = np.arange(0, X_LIMIT, RESOLUSION) self.l = np.arange(0, X_LIMIT, RESOLUSION) plt_t = self.fig.add_subplot(111) plt_t.set_ylim([-10, 50]) (self.line_t,) = plt_t.plot(x, self.data_t, "C1", label="Celsius") plt_h = plt_t.twinx() plt_h.set_ylim([0, 100]) (self.line_h,) = plt_h.plot(x, self.data_h, "C0", label="humidity %") plt_p = plt_t.twinx() plt_p.set_ylim([900, 1200]) (self.line_p,) = plt_p.plot(x, self.data_p, "C2", label="pressure hPa") h1, l1 = plt_t.get_legend_handles_labels() h2, l2 = plt_h.get_legend_handles_labels() h3, l3 = plt_p.get_legend_handles_labels() plt_t.legend(h1 + h2 + h3, l1 + l2 + l3, loc="upper left") self.ani = animation.FuncAnimation( self.fig, self.animate, self.l, interval=int(5000 * RESOLUSION), blit=False, ) toolbar = NavigationToolbar2Tk(canvas, self.root) canvas.get_tk_widget().pack(fill="both") button = tkinter.Button(master=self.root, text="Quit", command=self.quit) button.pack() def quit(self): self.root.quit() self.root.destroy() def animate(self, i): global cntt wbgt = 0 try: data = bme280.sample(bus, address, calibration_params) t = data.temperature h = data.humidity p = data.pressure #暑さ指数を計算 t2 = round(t,-1) h2 = round(h,-1) p2 = round(p,-1) if t2>33 and h2>80: wbgt = 33 elif t2>32 and h2>75: wbgt = 31 elif t2>31 and h2>70: wbgt = 30 elif t2>30 and h2>65: wbgt = 29 elif t2>29 and h2>60: wbgt = 28 if wbgt >= 28: message = ("\n部屋の温度が既定値を超えました" + "\n部屋の暑さ指数は" + str(wbgt) + "です") payload = {'message': message} headers = {'Authorization': 'Bearer ' + line_notify_token} line_notify = requests.post(line_notify_api, data=payload, headers=headers) sys.exit() date_t.append(data.temperature) date_h.append(data.humidity) date_p.append(data.pressure) cntt = cntt + 1 print(cntt) except OSError: return self.data_t = np.append(self.data_t[1:], t) self.line_t.set_ydata(self.data_t) # update the data_t. self.data_h = np.append(self.data_h[1:], h) self.line_h.set_ydata(self.data_h) # update the data_h. self.data_p = np.append(self.data_p[1:], p) self.line_p.set_ydata(self.data_p) # update the data_p. def run(self): for num in range(10): tkinter.mainloop() if __name__ == "__main__": r = requests.get('https://weather.yahoo.co.jp/weather/jp/39/7410.html') soup = BeautifulSoup(r.content, "html.parser") wc = soup.find(class_="forecastCity") ws = [i.strip() for i in wc.text.splitlines()] wl = [i for i in ws if i != ""] message = ("\n" + "高知中部:" + wl[0] + "\n" + wl[1] + "\n" + "最高気温:" + wl[2] + "\n"+ "最低気温:" + wl[3] + "\n" + "\n" + wl[18] + "\n" + wl[19] + "\n" + "最高気温:" + wl[20] + "\n" + "最低気温:" + wl[21] ) payload = {'message': message} headers = {'Authorization': 'Bearer ' + line_notify_token} line_notify = requests.post(line_notify_api, data=payload, headers=headers) cntt = 0 gui = GUI() gui.run() ```
# ラズベリーパイ画面 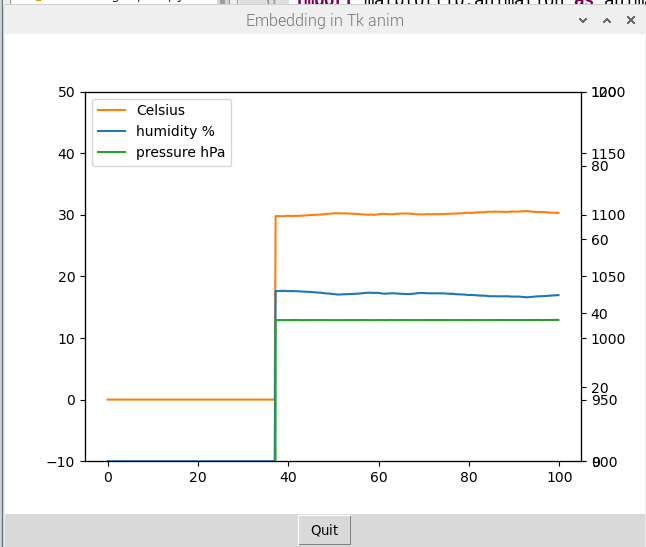 温度、湿度、気圧の変化をグラフに表示している。
必要となる物品 - raspi BME280 参考文献 - [室内の熱中症と暑さ指数(WBGT)](https://www.hmc.or.jp/news/wbgt.html)