yukinco が 2024年01月02日23時41分53秒 に編集
コメント無し
本文の変更
# Simple Web Camera Spresense、HDR カメラボード、W5500 Ether 拡張ボードを使ってシンプルな Web Camera を作ってみました。 今回のコードでは 192.168.1.240 にブラウザでアクセスすると Spresense の HDR カメラボードで撮影した JPG 画像データを表示します。 ## 部品 - Spresense Main Board - Spresense HDR Camera Board - W5500-Ether 拡張ボード ## 配線 画像のように HDR カメラボード、W5500 Ether 拡張ボードを Spresense Main Board に接続してください。 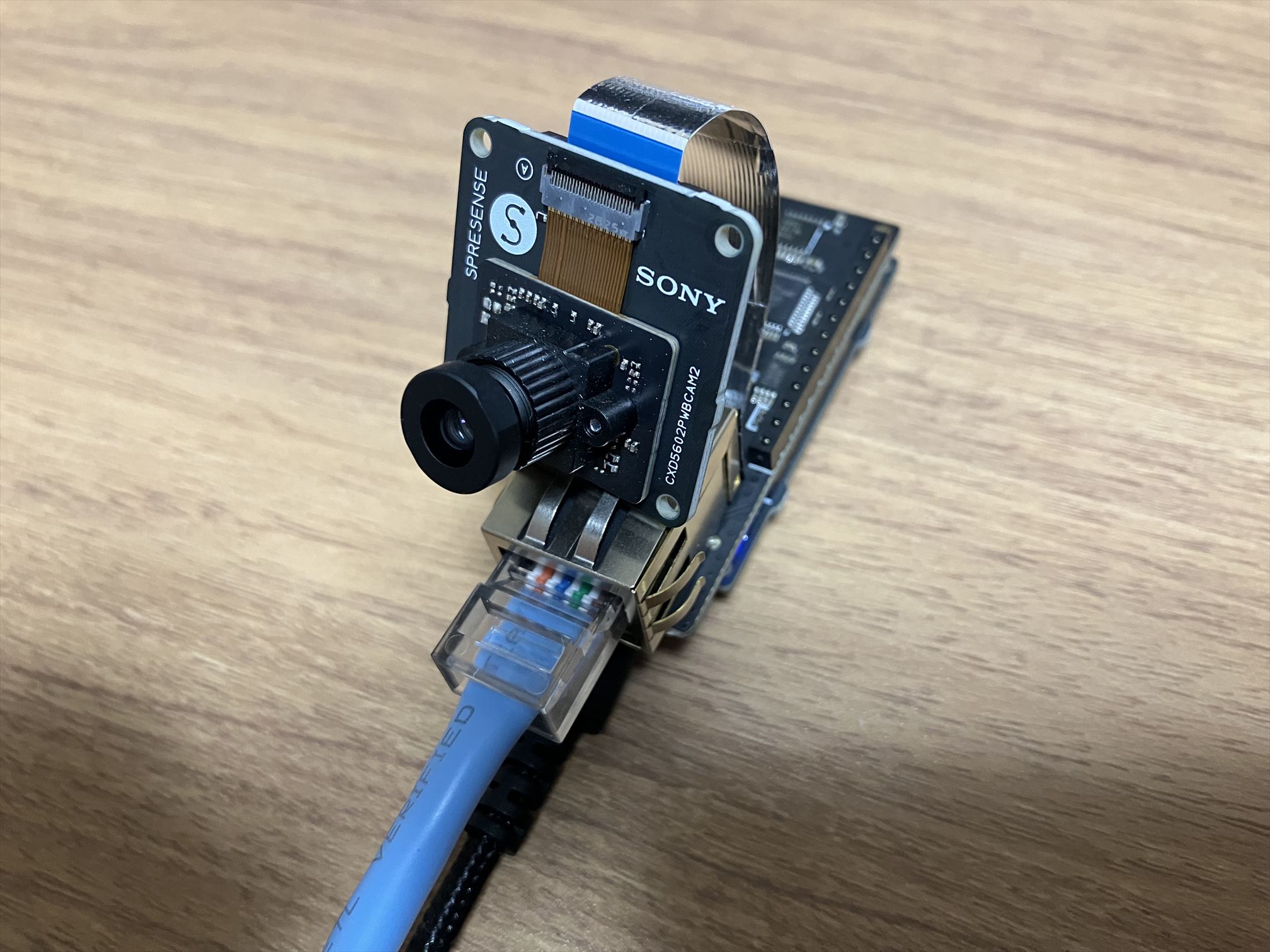 ## ソースコード src/Ethernet.h、src/M24C64.h についてはクレイン電子より提供されているサンプルコードそのままなので、割愛します。 (https://crane-elec.co.jp/products/vol-20/) ``` #include <SPI.h> #include <Camera.h> //Add-onボード用インクルード #include "src/Ethernet.h" #include "src/M24C64.h" #define BAUDRATE (115200) //Add-onボード用のSPIはSPI5となるため、本ライブラリ使用時は以下の定義が必要(socket.cpp内にて使用) //参考:https://developer.sony.com/develop/spresense/docs/arduino_developer_guide_ja.html#_spi_%E3%83%A9%E3%82%A4%E3%83%96%E3%83%A9%E3%83%AA #define USE_SPRESENSE_SPI5 byte mac[] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }; IPAddress ip(192, 168, 1, 240); // Initialize the Ethernet server library // with the IP address and port you want to use // (port 80 is default for HTTP): EthernetServer server(80); //Add-onボード搭載EEPROMへのアクセス用 M24C64 eep; void printErrorForCamera(enum CamErr err) { Serial.print("Error: "); switch (err) { case CAM_ERR_NO_DEVICE: Serial.println("No Device"); break; case CAM_ERR_ILLEGAL_DEVERR: Serial.println("Illegal device error"); break; case CAM_ERR_ALREADY_INITIALIZED: Serial.println("Already initialized"); break; case CAM_ERR_NOT_INITIALIZED: Serial.println("Not initialized"); break; case CAM_ERR_NOT_STILL_INITIALIZED: Serial.println("Still picture not initialized"); break; case CAM_ERR_CANT_CREATE_THREAD: Serial.println("Failed to create thread"); break; case CAM_ERR_INVALID_PARAM: Serial.println("Invalid parameter"); break; case CAM_ERR_NO_MEMORY: Serial.println("No memory"); break; case CAM_ERR_USR_INUSED: Serial.println("Buffer already in use"); break; case CAM_ERR_NOT_PERMITTED: Serial.println("Operation not permitted"); break; default: break; } }
void cameraCallback(CamImage img) { // do nothing }
bool isI2C(uint8_t address) { Wire.beginTransmission(address); if (Wire.endTransmission() == 0) { return true; } return false; } void initializeW5500Ether() { digitalWrite(21, LOW); //W5500_Eth RESET# = HIGH delay(500); digitalWrite(21, HIGH); //W5500_Eth RESET# = HIGH Serial.println("W5500 reset done."); Ethernet.init(19); // use I2S_DIN pin for W5500 CS pin } void getMacAddressFromEEPROM() { // I2C device scan Serial.print(F("I2C Devices(0x) : ")); Wire.begin(); // I2C spec. have reserved address!! these scanning escape it address. for (uint8_t ad = 0x08; ad < 0x77; ad++) { if (isI2C(ad)) { Serial.print(ad, HEX); Serial.write(' '); } } Serial.write('\n'); //EEPROM MAC ADDRESS read eep.init(0x57); Serial.println("MAC read from on board eeprom."); for (int i = 0; i < 6; i++) { mac[i] = eep.read(i); Serial.print(mac[i],HEX); Serial.print(":"); } Serial.println(""); } void setup() { CamErr err; Serial.begin(115200); while (!Serial) { ; // wait for serial port to connect. Needed for native USB port only } Serial.println("Ethernet WebServer Example"); // Intialize Camera Serial.println("Prepare camera"); err = theCamera.begin(); if (err != CAM_ERR_SUCCESS) { printErrorForCamera(err); } // Start Streaming Serial.println("Start streaming"); err = theCamera.startStreaming(true); if (err != CAM_ERR_SUCCESS) { printErrorForCamera(err); } // Auto white balance configuration Serial.println("Set Auto white balance parameter"); err = theCamera.setAutoWhiteBalanceMode(CAM_WHITE_BALANCE_DAYLIGHT); if (err != CAM_ERR_SUCCESS) { printErrorForCamera(err); } // Set parameters about still picture. Serial.println("Set still picture format"); err = theCamera.setStillPictureImageFormat( CAM_IMGSIZE_QQVGA_H, CAM_IMGSIZE_QQVGA_V, CAM_IMAGE_PIX_FMT_JPG); if (err != CAM_ERR_SUCCESS) { printErrorForCamera(err); } // W5500-Ether の初期化 initializeW5500Ether(); // W5500-Ether用のMACアドレス取得処理 getMacAddressFromEEPROM(); // start the Ethernet connection and the server: Ethernet.begin(mac, ip); // Check for Ethernet hardware present if (Ethernet.hardwareStatus() == EthernetNoHardware) { Serial.println("Ethernet shield was not found. Sorry, can't run without hardware. :("); while (true) { delay(1); // do nothing, no point running without Ethernet hardware } } // Link Status が落ち着くまで待つ while (Ethernet.linkStatus() == LinkOFF) { delay(1000); // 1000ms = 1s Serial.println("Ethernet cable is not connected."); } // start the server server.begin(); Serial.print("server is at "); Serial.println(Ethernet.localIP()); } void loop() { // listen for incoming clients EthernetClient client = server.available(); if (client) { Serial.println("new client"); // an http request ends with a blank line bool currentLineIsBlank = true; while (client.connected()) { if (client.available()) { char c = client.read(); Serial.write(c); // if you've gotten to the end of the line (received a newline // character) and the line is blank, the http request has ended, // so you can send a reply if (c == '\n' && currentLineIsBlank) { // send a standard http response header client.println("HTTP/1.1 200 OK"); client.println("Content-Type: image/jpeg"); client.println("Connection: close"); // the connection will be closed after completion of the response client.println(); CamImage img = theCamera.takePicture(); if (img.isAvailable()) { client.write(img.getImgBuff(), img.getImgSize()); } break; } if (c == '\n') { // you're starting a new line currentLineIsBlank = true; } else if (c != '\r') { // you've gotten a character on the current line currentLineIsBlank = false; } } } // give the web browser time to receive the data delay(1); // close the connection: client.stop(); Serial.println("client disconnected"); } } ```